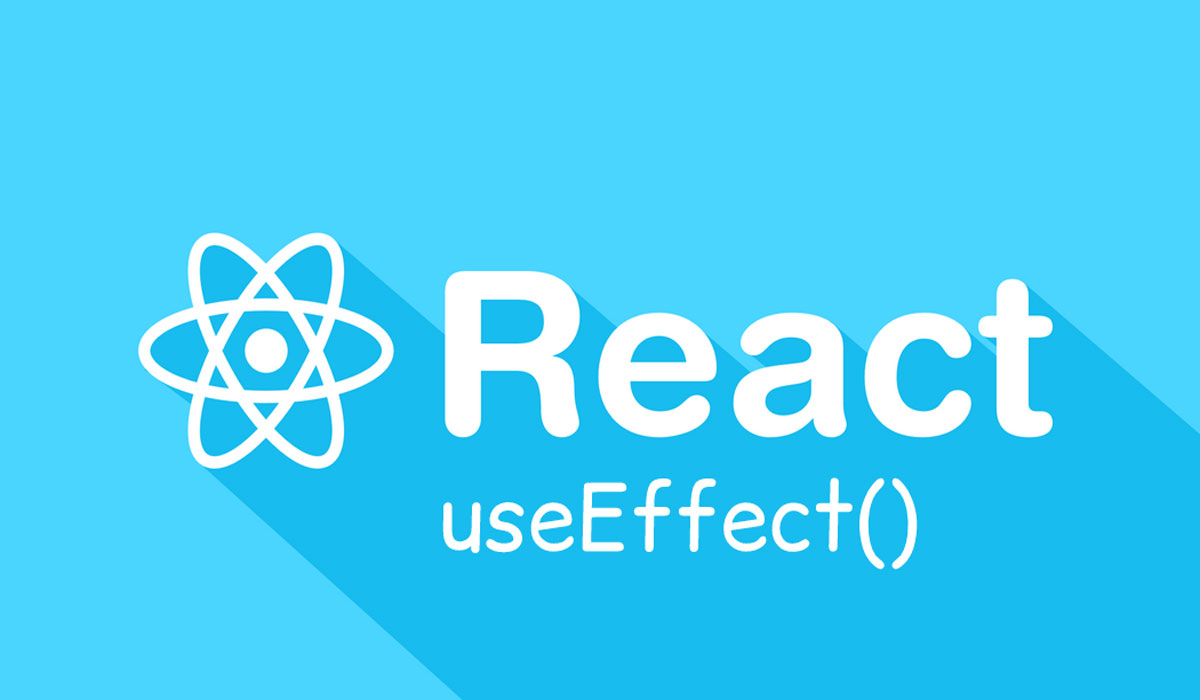
React’s useEffect
hook is one of the most powerful and commonly used hooks in functional components. It allows you to perform side effects, such as fetching data, updating the DOM, or subscribing to events, in a declarative and efficient way. In this article, we’ll dive deep into the useEffect
hook, explore its usage, and provide practical examples to help you master it. This guide is also optimized for SEO to help you find the information you need quickly.
What is the useEffect
Hook?
The useEffect
hook is a built-in React hook that enables you to perform side effects in functional components. It serves as a replacement for lifecycle methods like componentDidMount
, componentDidUpdate
, and componentWillUnmount
in class components.
Why Use useEffect
?
- Declarative Code:
useEffect
allows you to express side effects in a declarative way, making your code easier to read and maintain. - Functional Components: With
useEffect
, you can use side effects in functional components without needing to convert them to class components. - Lifecycle Management: It simplifies lifecycle management by consolidating multiple lifecycle methods into a single hook.
Basic Syntax of useEffect
The useEffect
hook takes two arguments:
- A function that contains the side effect logic.
- An optional dependency array that controls when the effect runs.
useEffect(() => {
// Side effect logic here
return () => {
// Cleanup logic (optional)
};
}, [dependencies]);
Common Use Cases for useEffect
Let’s explore the most common use cases for the useEffect
hook with examples.
1. Fetching Data
One of the most common use cases for useEffect
is fetching data from an API.
import React, { useState, useEffect } from 'react';
function DataFetchingComponent() {
const [data, setData] = useState([]);
useEffect(() => {
fetch('https://jsonplaceholder.typicode.com/posts')
.then((response) => response.json())
.then((data) => setData(data))
.catch((error) => console.error('Error fetching data:', error));
}, []); // Empty dependency array means this runs once on mount
return (
<div>
<h1>Posts</h1>
<ul>
{data.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
</div>
);
}
export default DataFetchingComponent;
In this example, the useEffect
hook fetches data from an API when the component mounts.
2. Updating the Document Title
You can use useEffect
to update the document title dynamically.
import React, { useState, useEffect } from 'react';
function DocumentTitleUpdater() {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `Count: ${count}`;
}, [count]); // Runs whenever `count` changes
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
export default DocumentTitleUpdater;
This example updates the document title whenever the count
state changes.
3. Subscribing to Events
You can use useEffect
to subscribe to events, such as window resizing.
import React, { useState, useEffect } from 'react';
function WindowSizeTracker() {
const [windowSize, setWindowSize] = useState({
width: window.innerWidth,
height: window.innerHeight,
});
useEffect(() => {
const handleResize = () => {
setWindowSize({
width: window.innerWidth,
height: window.innerHeight,
});
};
window.addEventListener('resize', handleResize);
return () => {
window.removeEventListener('resize', handleResize);
};
}, []); // Empty dependency array means this runs once on mount and cleans up on unmount
return (
<div>
<p>Width: {windowSize.width}px</p>
<p>Height: {windowSize.height}px</p>
</div>
);
}
export default WindowSizeTracker;
This example tracks the window size and updates the state whenever the window is resized.
4. Cleanup with useEffect
The useEffect
hook can return a cleanup function to perform cleanup tasks, such as unsubscribing from events or canceling API requests.
/v
import React, { useState, useEffect } from ‘react’;
function TimerComponent() {
const [time, setTime] = useState(0);
useEffect(() => {
const interval = setInterval(() => {
setTime((prevTime) => prevTime + 1);
}, 1000);
return () => {
clearInterval(interval); // Cleanup the interval on unmount
};
}, []); // Empty dependency array means this runs once on mount
return (
<div>
<p>Time: {time} seconds</p>
</div>
);
}
export default TimerComponent;
This example sets up a timer and cleans it up when the component unmounts.
Advanced Usage of useEffect
Conditional Execution with Dependencies
The dependency array controls when the effect runs. If the array is empty, the effect runs only once on mount. If it contains values, the effect runs whenever those values change.
useEffect(() => {
console.log('Count has changed:', count);
}, [count]); // Runs whenever `count` changes
Multiple useEffect
Hooks
You can use multiple useEffect
hooks in a single component to separate concerns.
useEffect(() => {
// Logic for side effect 1
}, [dependency1]);
useEffect(() => {
// Logic for side effect 2
}, [dependency2]);
Avoiding Infinite Loops
Be careful when updating state inside useEffect
without proper dependencies, as it can cause infinite loops.
useEffect(() => {
setCount(count + 1); // This will cause an infinite loop
}, [count]); // `count` changes every time the effect runs
To avoid this, ensure that state updates are conditional or use a different dependency.
Best Practices for Using useEffect
- Keep Effects Focused: Each
useEffect
should handle a single side effect to keep your code clean and maintainable. - Use Cleanup Functions: Always clean up subscriptions, timers, or event listeners to avoid memory leaks.
- Optimize Dependencies: Only include dependencies that are necessary for the effect to run.
- Avoid Unnecessary Renders: Use
useMemo
oruseCallback
to memoize values or functions that are passed as dependencies.
Practical Example: Building a Data Fetching Hook
Let’s create a custom hook for fetching data using useEffect
.
Step 1: Create the Custom Hook
import { useState, useEffect } from 'react';
function useFetch(url) {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch(url);
const result = await response.json();
setData(result);
} catch (error) {
setError(error);
} finally {
setLoading(false);
}
};
fetchData();
}, [url]); // Re-run effect when `url` changes
return { data, loading, error };
}
export default useFetch;
/v
Step 2: Use the Custom Hook in a Component
import React from 'react';
import useFetch from './useFetch';
function DataFetchingComponent() {
const { data, loading, error } = useFetch('https://jsonplaceholder.typicode.com/posts');
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
return (
<div>
<h1>Posts</h1>
<ul>
{data.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
</div>
);
}
export default DataFetchingComponent;
Conclusion
The useEffect
hook is an essential tool for managing side effects in React functional components. Whether you’re fetching data, updating the DOM, or subscribing to events, useEffect
provides a clean and efficient way to handle these tasks. By following best practices and understanding its advanced usage, you can write more robust and maintainable React applications.