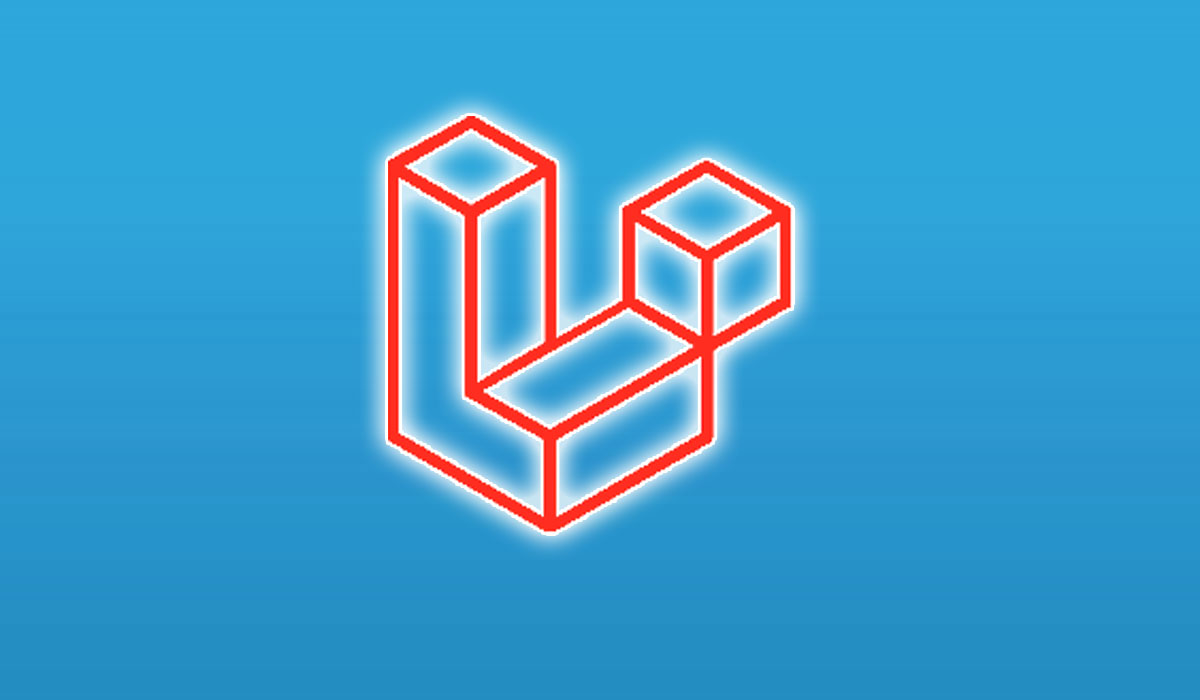
Laravel, one of the most popular PHP frameworks, is renowned for its elegant syntax, robust features, and developer-friendly tools. Among its many capabilities, Laravel provides powerful tools for working with databases, including aggregators. Aggregators are essential for performing calculations on datasets, such as counting, summing, averaging, and more. In this article, we’ll dive deep into Laravel aggregators, explore their usage, and provide practical examples to help you optimize your database queries.
What Are Laravel Aggregators?
Aggregators in Laravel are methods provided by the Eloquent ORM and Query Builder that allow you to perform aggregate operations on your database tables. These operations include:
- Counting the number of records.
- Summing up values in a column.
- Averaging values in a column.
- Finding the minimum or maximum value in a column.
Aggregators are particularly useful when you need to extract summarized information from large datasets without fetching all the records.
Why Use Aggregators in Laravel?
- Performance Optimization: Aggregators allow you to offload calculations to the database, reducing the amount of data transferred to your application and improving performance.
- Simplified Code: Instead of manually iterating through records to calculate totals or averages, you can use built-in methods to achieve the same result with minimal code.
- Database Agnostic: Laravel’s aggregators work seamlessly across different database systems (MySQL, PostgreSQL, SQLite, etc.), ensuring consistency in your application.
Common Laravel Aggregators
Let’s explore the most commonly used aggregators in Laravel with examples.
1. count()
The count()
method returns the total number of records that match a query.
$totalUsers = DB::table('users')->count(); echo "Total Users: " . $totalUsers;
This query will return the total number of users in the users
table.
2. sum()
The sum()
method calculates the total sum of a numeric column.
$totalRevenue = DB::table('orders')->sum('amount'); echo "Total Revenue: $" . $totalRevenue;
This query calculates the total revenue from the orders
table by summing up the amount
column.
3. avg()
The avg()
method calculates the average value of a numeric column.
$averageOrderValue = DB::table('orders')->avg('amount'); echo "Average Order Value: $" . $averageOrderValue;
This query computes the average value of orders in the orders
table.
4. min()
and max()
The min()
and max()
methods retrieve the minimum and maximum values of a column, respectively.
$minOrder = DB::table('orders')->min('amount'); $maxOrder = DB::table('orders')->max('amount'); echo "Minimum Order: $" . $minOrder . ", Maximum Order: $" . $maxOrder;
These queries fetch the smallest and largest order amounts from the orders
table.
5. groupBy()
with Aggregators
You can combine groupBy()
with aggregators to perform calculations on grouped data.
$revenueByUser = DB::table('orders') ->select('user_id', DB::raw('SUM(amount) as total_revenue')) ->groupBy('user_id') ->get(); foreach ($revenueByUser as $revenue) { echo "User ID: " . $revenue->user_id . ", Total Revenue: $" . $revenue->total_revenue . "\n"; }
This query groups orders by user_id
and calculates the total revenue for each user.
Advanced Aggregator Usage
Conditional Aggregations
You can use the when()
method to conditionally apply aggregators.
$revenue = DB::table('orders') ->when($request->has('status'), function ($query) use ($request) { return $query->where('status', $request->input('status')); }) ->sum('amount');
This query sums up the amount
column only if a status
parameter is provided in the request.
Combining Multiple Aggregators
You can combine multiple aggregators in a single query.
$stats = DB::table('orders') ->select( DB::raw('COUNT(*) as total_orders'), DB::raw('SUM(amount) as total_revenue'), DB::raw('AVG(amount) as average_revenue') ) ->first(); echo "Total Orders: " . $stats->total_orders . "\n"; echo "Total Revenue: $" . $stats->total_revenue . "\n"; echo "Average Revenue: $" . $stats->average_revenue . "\n";
This query retrieves the total number of orders, total revenue, and average revenue in a single query.
Optimizing Aggregator Queries
Indexing Columns
Ensure that columns used in aggregator queries (e.g., amount
, user_id
) are indexed. Indexing improves query performance by reducing the time it takes to search and retrieve data.
CREATE INDEX idx_amount ON orders (amount);
Caching Aggregator Results
For frequently accessed aggregator queries, consider caching the results to reduce database load.
$totalRevenue = Cache::remember('total_revenue', 3600, function () { return DB::table('orders')->sum('amount'); });
This code caches the total revenue for one hour.
Using Raw Expressions
For complex calculations, you can use raw expressions with DB::raw()
.
$totalRevenue = DB::table('orders') ->select(DB::raw('SUM(amount * tax_rate) as total_revenue')) ->first();
This query calculates the total revenue by multiplying the amount
and tax_rate
columns.
Practical Example: Building a Sales Dashboard
Let’s build a simple sales dashboard using Laravel aggregators.
Step 1: Define Routes
Route::get('/dashboard', [DashboardController::class, 'index']);
Step 2: Create Controller
namespace App\Http\Controllers; use Illuminate\Support\Facades\DB; class DashboardController extends Controller { public function index() { $totalRevenue = DB::table('orders')->sum('amount'); $averageOrderValue = DB::table('orders')->avg('amount'); $totalOrders = DB::table('orders')->count(); return view('dashboard', compact('totalRevenue', 'averageOrderValue', 'totalOrders')); } }
Step 3: Create Blade View
<!DOCTYPE html> <html> <head> <title>Sales Dashboard</title> </head> <body> <h1>Sales Dashboard</h1> <p>Total Revenue: ${{ $totalRevenue }}</p> <p>Average Order Value: ${{ $averageOrderValue }}</p> <p>Total Orders: {{ $totalOrders }}</p> </body> </html>
Conclusion
Laravel aggregators are powerful tools for performing calculations on your database tables efficiently. By leveraging methods like count()
, sum()
, avg()
, min()
, and max()
, you can simplify your code and optimize your application’s performance. Whether you’re building a sales dashboard or analyzing user data, aggregators are an essential part of your Laravel toolkit.
By following the best practices outlined in this article, such as indexing columns, caching results, and using raw expressions, you can take full advantage of Laravel’s aggregator capabilities and build high-performance applications.