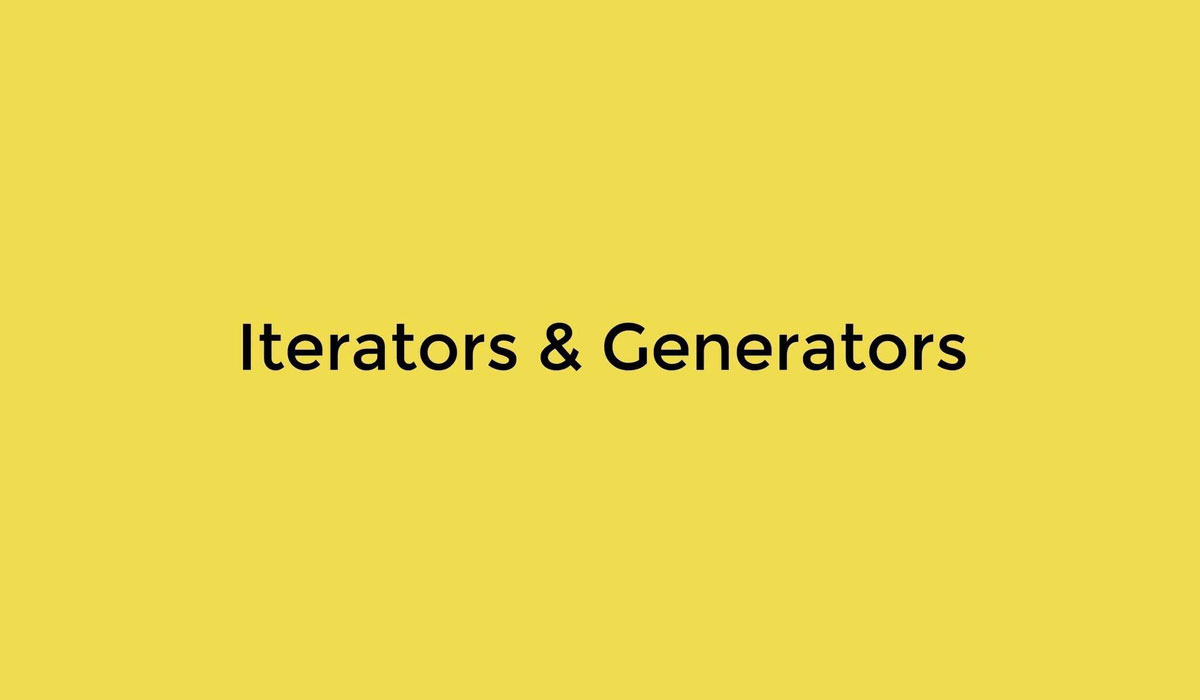
Generators and Iterators: The Art of Lazy Evaluation in Modern Programming
Imagine you’re at an all-you-can-eat buffet. Instead of piling everything onto your plate at once (and risking waste), you take small portions as needed. This is the essence of generators and iterators in programming: they let you consume data on-demand rather than loading it all into memory upfront. In this deep dive, we’ll explore these concepts through metaphors, code examples, and real-world analogies.
Iterators: The Universal Remote for Data
An iterator is like a bookmark in a novel. It remembers where you left off and knows how to get the next page. In technical terms, an iterator is an object that implements:
- A
next()
method (JavaScript) or__next__()
(Python) - A protocol to signal when there’s nothing left to read
Python Iterator Example
class Countdown:
def __init__(self, start):
self.current = start
def __iter__(self):
return self
def __next__(self):
if self.current <= 0:
raise StopIteration
num = self.current
self.current -= 1
return num
# Usage
for number in Countdown(5):
print(number) # Outputs 5,4,3,2,1
This iterator works like an advent calendar – each day you open a new compartment (call next()
) until there’s nothing left.
JavaScript Iterator Example
function createCountdown(start) {
let current = start;
return {
[Symbol.iterator]: function() {
return this;
},
next: function() {
if (current <= 0) {
return { done: true };
}
return { value: current--, done: false };
}
};
}
// Usage
for (const num of createCountdown(5)) {
console.log(num); // 5,4,3,2,1
}
Generators: The Data Factory Assembly Line
If iterators are bookmarks, generators are the printing press that creates books on demand. They let you generate values lazily using special syntax.
Python Generator Example
def infinite_sequence():
num = 0
while True:
yield num
num += 1
# Usage
gen = infinite_sequence()
print(next(gen)) # 0
print(next(gen)) # 1
# Could theoretically run forever
This generator is like a waterwheel – it produces a new value each time you push it (call next()
), but doesn’t store all previous values.
JavaScript Generator Example
function* infiniteSequence() {
let num = 0;
while (true) {
yield num++;
}
}
// Usage
const gen = infiniteSequence();
console.log(gen.next().value); // 0
console.log(gen.next().value); // 1
Key Differences: Iterators vs Generators
Feature | Iterators | Generators |
---|---|---|
Memory Usage | Manual management | Automatic suspension |
Syntax | Class-based | Function with yield |
State | Explicit | Implicit |
Complexity | Higher | Lower |
Real-World Use Cases
1. Large File Processing
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line.strip()
# Process 10GB file without loading to RAM
for line in read_large_file('huge.log'):
process_line(line)
Like reading a scroll one paragraph at a time instead of unrolling the entire thing.
2. Infinite Sequences
function* uniqueIdGenerator(prefix) {
let count = 0;
while (true) {
yield `${prefix}-${count++}`;
}
}
const userIdGen = uniqueIdGenerator('user');
console.log(userIdGen.next().value); // user-0
console.log(userIdGen.next().value); // user-1
Like a never-ending roll of lottery tickets – you only print the next number when needed.
Common Pitfalls and Best Practices
- Memory Leaks: Always close generators when done (Python’s
gen.close()
) - Reusability: Generators exhaust themselves – like a soda can you can’t refill
- Error Handling: Use try/finally blocks in generators for cleanup
Python Generator Cleanup
def sensor_data():
try:
while True:
yield read_sensor()
finally:
cleanup_sensor()
The Iterator Protocol: Behind the Scenes
When you use a for
loop, here’s what happens:
- Calls
iter()
on the object - Repeatedly calls
next()
- Handles
StopIteration
/done: true
It’s like having a personal assistant who:
- 1. Finds the book (iterable)
- 2. Turns to the first page (iterator)
- 3. Keeps turning pages until “The End”
Advanced Patterns
Generator Pipelines
def parse_numbers(lines):
for line in lines:
yield float(line.strip())
def square(numbers):
for n in numbers:
yield n ** 2
# Create pipeline
lines = open('data.txt')
numbers = parse_numbers(lines)
squares = square(numbers)
print(sum(squares)) # Process entire stream efficiently
Like an assembly line where each workstation (generator) processes items as they arrive.
Async Generators (Python 3.6+)
async def stream_sensor_data():
while True:
data = await fetch_sensor_async()
yield data
async for reading in stream_sensor_data():
process(reading)
Like having a waiter who brings courses only when you’re ready for them.
Conclusion: The Power of Lazy Evaluation
Generators and iterators are like the difference between:
- Streaming a movie vs downloading the entire file
- Using a water fountain vs buying bottled water
- Reading a book page-by-page vs memorizing the whole text
By mastering these concepts, you’ll write more memory-efficient code that can handle infinite data streams and complex processing pipelines with elegance. Remember: in programming as in life, sometimes lazy is smart!